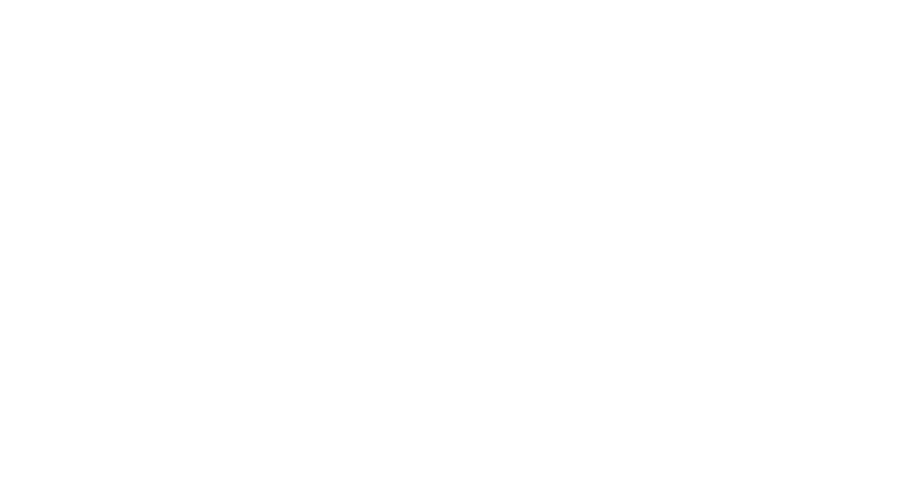
If you are a beginner in PHP and your manager assigned you a task to create responsive + interactive web form to upload image with certain conditions & Validations, then you came to the right place. Simple HTML pages are allowed to use PHP code in it and that PHP code can interact with the server to upload the files. We will try to explain all image upload conditions by showing you the proper code and interface GUI.
Check before we start:
1. php.ini file settings: Before we start we need to check our php.ini file having a maximum permitted size of files that can be uploaded is stated as upload_max_filesize. Settings should look like below:
file_uploads = On
upload_max_filesize = 150M
post_max_size = 150M
2. Create Uploads folder: Create a folder named uploads along with your code where your images will be stored. This directory must have the read write permission.
Now let’s start coding
Step 1: Create index.html which will have a webform
Create a form in this html page, which use method attribute as “post” and enctype should be “multipart/form-data”. It specifies which content type should be used, without enctype file upload will not work. One Input file and one submit button is required. Your Code will look like below
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css">
</head>
<body>
<div class="my-5 mx-auto" style="max-width: 320px;">
<h2>PHP File Uploader</h2>
<hr>
<!---method must be post and enctype must be multipart/form-data-->
<form action="upload.php" method="post" enctype="multipart/form-data">
<div class="form-group">
<label for="fileToUpload">Select image file to upload</label>
<input type="file" name="fileToUpload" id="fileToUpload" class="form-control-file"> <!--input type must be file -->
</div>
<hr>
<input type="submit" value="Upload Image" name="submit" class="btn btn-primary">
</form>
</div>
</body>
</html>
we are using bootstrap 4 css to make it intreactive, Your Output will be like below:
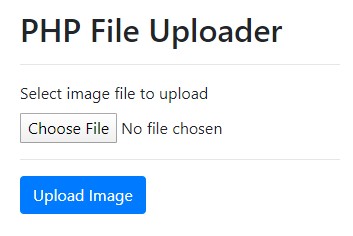
Step 2: Create upload.php for PHP coding
PHP has a global variable $_FILES.This keeps all information of uploaded files like image name, image size, image path, etc in the form of an array.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css">
</head>
<body>
<div class="my-5 mx-auto" style="max-width: 320px;">
<!-- PHP Code Start -->
<?php
$target_dir = "uploads/";
$target_file = $target_dir . basename($_FILES["fileToUpload"]["name"]);
$uploadOk = 1;
$imageFileType = strtolower(pathinfo($target_file,PATHINFO_EXTENSION));
// Check if image file is a actual image or fake image
if(isset($_POST["submit"])) {
$check = getimagesize($_FILES["fileToUpload"]["tmp_name"]);
if($check !== false) {
echo '<img src="'.$target_file.'" class="img-fluid img-thumbnail mb-3" />';
$uploadOk = 1;
} else {
echo "<div class='alert alert-danger'>File is not an image.</div>";
$uploadOk = 0;
}
}
// Check if file already exists
if (file_exists($target_file)) {
echo "<div class='alert alert-danger'>Sorry, file already exists.</div>";
$uploadOk = 0;
}
// Check file size
if ($_FILES["fileToUpload"]["size"] > 500000) {
echo "<div class='alert alert-danger'>Sorry, your file is too large.</div>";
$uploadOk = 0;
}
// Allow certain file formats
if($imageFileType != "jpg" && $imageFileType != "png" && $imageFileType != "jpeg"
&& $imageFileType != "gif" ) {
echo "<div class='alert alert-danger'>Sorry, only JPG, JPEG, PNG & GIF files are allowed.</div>";
$uploadOk = 0;
}
// Check if $uploadOk is set to 0 by an error
if ($uploadOk == 0) {
echo "<div class='alert alert-danger'>Sorry, your file was not uploaded.</div>";
// if everything is ok, try to upload file
} else {
if (move_uploaded_file($_FILES["fileToUpload"]["tmp_name"], $target_file)) {
echo "<div class='alert alert-success'>The file ". basename( $_FILES["fileToUpload"]["name"]). " has been uploaded.</div>";
} else {
echo "<div class='alert alert-danger'>Sorry, there was an error uploading your file.</div>";
}
}
?>
</div>
</body>
</html>
Your Output will be like below:
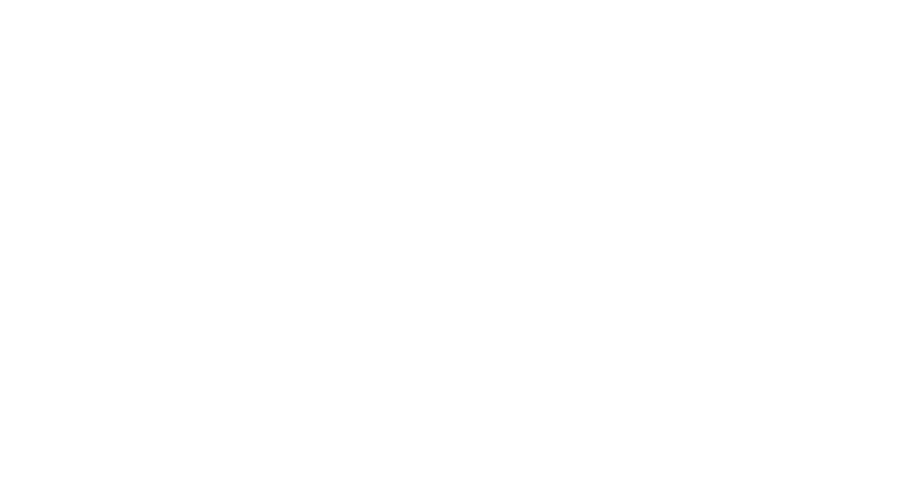
Now we will explain from this PHP code what all functionality covered
#1. Check your upload folder is having the file you upload
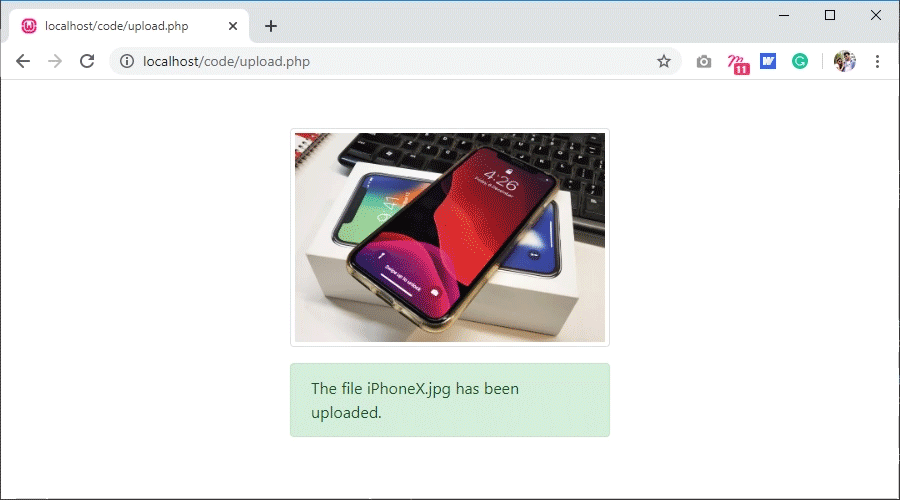
#2. If the file is already exist
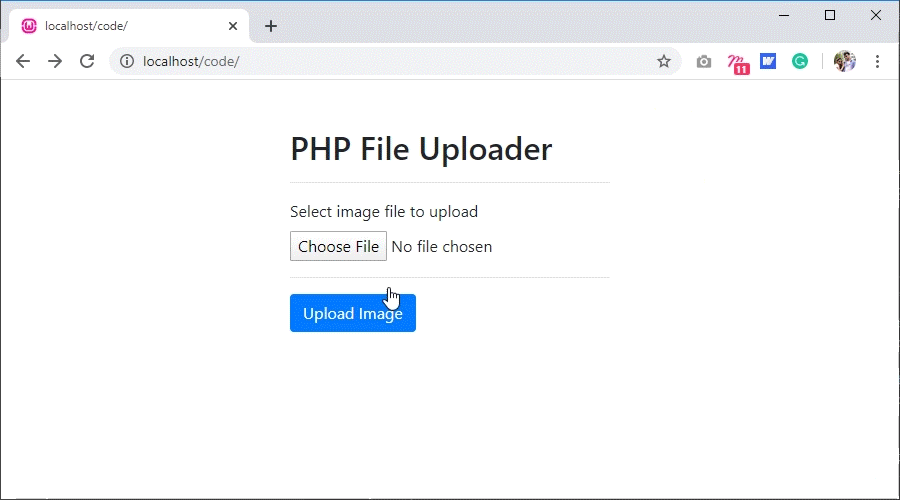
#3. Check if the file is an image
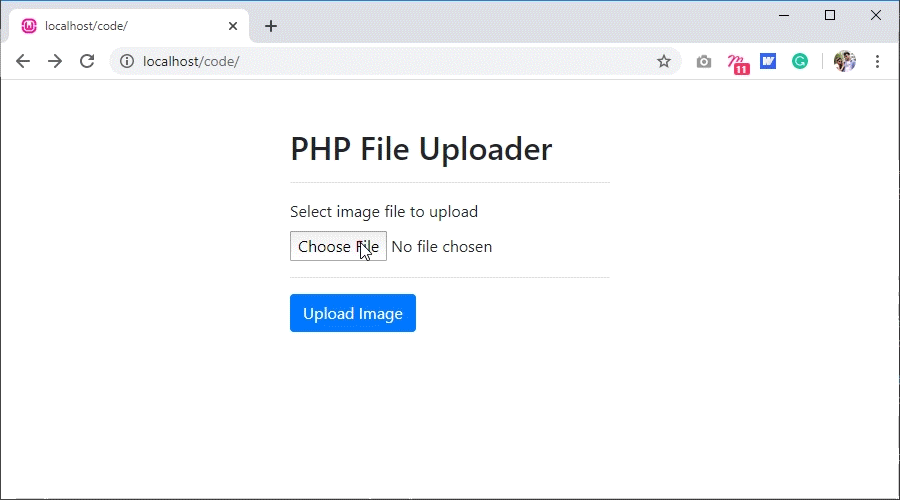
#4. Check if the file is not a large file
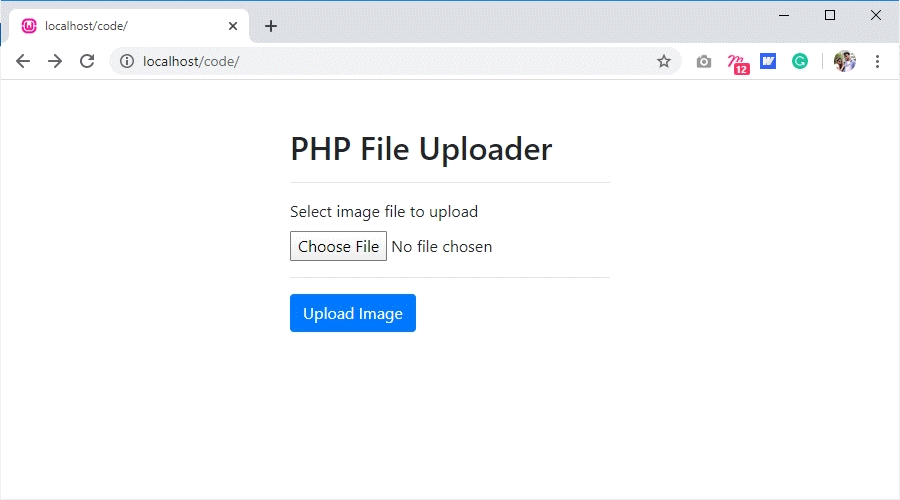
#5. Check if the file is JPG, JPEG, GIF, PNG only
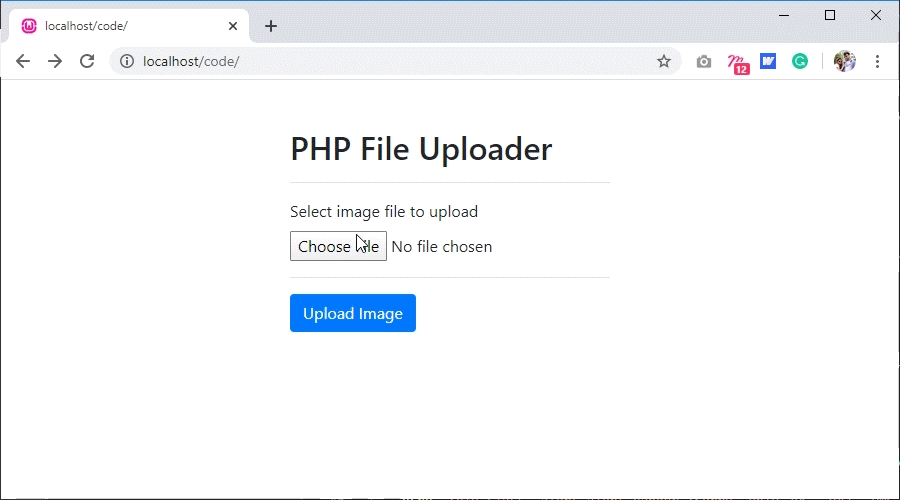
You can download the code from Github
2 replies on “Basic PHP code for Image upload”
please also write an article for php functionality like pagination, sorting ,json etc
Hi Guys do you agree?