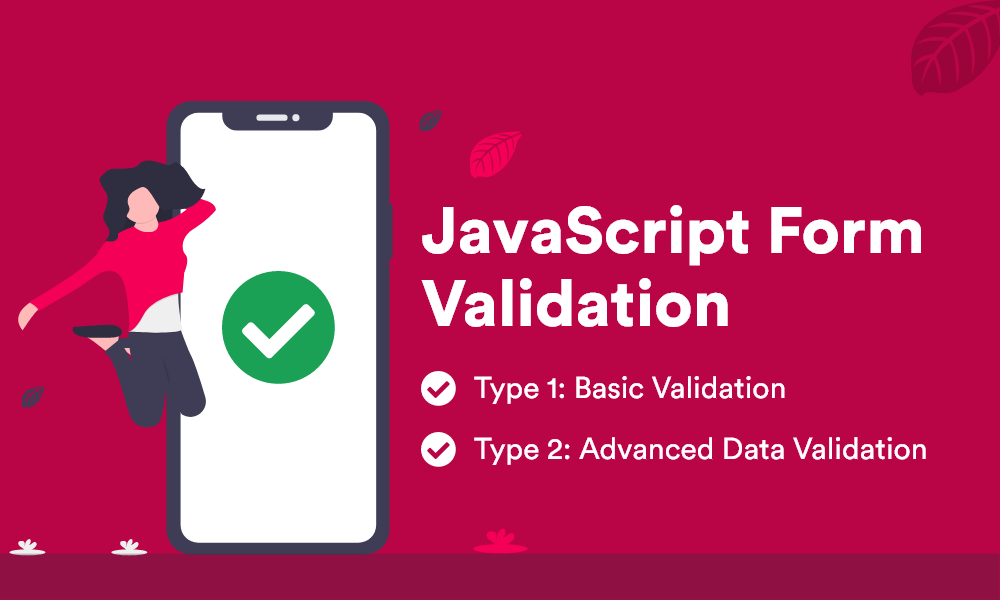
We will start with very basic form validation through JavaScript, it will be a collection for a few form fields and a few types of validations. To collect user’s information such as Name, Email Address, Phone Numbers, Password, etc we create web forms and To collect correct information from the website users, we need to validate the form data before sending it to server-side code or our databases. JavaScript provides faster client-side form validation than server-side validation does. Server-side validation requires more time first occurring on the server, which requires the user’s input to be submitted and sent to the server before validation occurs. Therefore, client-side validation helps to create a better user experience.
Here is the step by step procedure to validate a web form.
Step 1: Create index.html file with user input fields and a submit button where “onsubmit” attribute fires when the form is submitted and validateForm() is a user-defined javascript method where we will write code for validation on input fields.
<!DOCTYPE html>
<html>
<body>
<form action="index.html" method="post" onsubmit="return validateForm()" name="myForm">
<p><input type="text" name="fname" placeholder="Full Name"></p>
<p><input type="text" name="email" placeholder="Email"></p>
<p><input type="password" name="password" placeholder="Password"></p>
<p><input type="text" name="phone" placeholder="Phone"></p>
<p><input type="submit" value="Submit"></p>
</form>
</body>
</html>
Step 2: Add JavaScript code at the head section of index.html with a script tag.
<script>
function validateForm() {
var fname = document.forms["myForm"]["fname"].value;
var email = document.forms["myForm"]["email"].value;
var password = document.forms["myForm"]["password"].value;
var phone = document.forms["myForm"]["phone"].value;
//check for empty input fields
if (fname == "") {
alert("Name must be entered.");
fname.focus;
return false;
}
if (email == "") {
alert("Email must be entered.");
email.focus;
return false;
}
if (password == "") {
alert("Password must be entered.");
password.focus;
return false;
}
if (phone == "") {
alert("Phone Number must be entered.");
phone.focus;
return false;
}
}
</script>
Output
See the Pen Type 1: JavaScript Form Validation for Beginners – CreativeAlive Team (Akshma) by Ravi Joon (@ravijoon) on CodePen.
Step 3: Advanced Data Form Validation
<script>
function validateForm() {
var fname = document.forms["myForm"]["fname"].value;
var email = document.forms["myForm"]["email"].value;
var phone = document.forms["myForm"]["phone"].value;
//check if name is not alphabets
var check_alpha = /^[a-zA-Z]+$/;
if (!check_alpha.test(fname)) {
alert('Please provide a valid name.Allowed alphabets only!');
fname.focus;
return false;
}
//check if email is not valid
var check_email = /^([a-zA-Z0-9_\.\-])+\@(([a-zA-Z0-9\-])+\.)+([a-zA-Z0-9]{2,4})+$/;
if (!check_email.test(email)) {
alert('Please provide a valid email address.');
email.focus;
return false;
}
//check if phone is not a number
var numbers = /^[-+]?[0-9]+$/;
if (!numbers.test(phone)) {
alert('Please provide a valid phone number.Allowed numbers only!');
phone.focus;
return false;
}
}
</script>
Output
See the Pen Type 2: JavaScript Form Validation for Beginners – CreativeAlive Team (Akshma) by Ravi Joon (@ravijoon) on CodePen.
Stay connect with us for more tasteful tutorials and
If you find it great please like/clap, share and subscribe me on YouTube 🙂
https://www.youtube.com/user/ravijoon52
